Rule-Based Data Filtering Using LINQ
IMPORTANT Due to limitations in the current version of Entity Framework the FlexSource technology is not supported in Data Filtering feature of Code Effects. Please contact us if you are going to support Code Effects in your own LINQ provider and need our help.
Besides business rules management, Code Effects engine provides a feature that is unique not only to the business rules industry but to .NET LINQ programming as well. It's called Rule-Based Data Filtering, and it can be used with any LINQ provider as long as it supports common operators, such as contains, less than, is null, and so on. You can try this feature by using our live demo.
A filter is nothing more than a business rule attached to a data selection query. It's safe to say that rule-based data filtering is a logical "side effect" of business rules.
You are already familiar with SQL filters if you have ever created a statement that uses a where clause. Although SQL provides an extremely powerful filtration mechanism, the problem is that access to that power is limited to people who are fluent in SQL. To combat this limitation and allow end users to define their own search criteria, applications usually include search forms.
A typical search form contains a single text box, a Go button, and a link that takes a user to an advanced search form. The text box is usually smart enough to recognize a proprietary syntax that the user can use to filter the search results. For example, a phrase in quotes would return all items that contain that exact phrase, while that same phrase without quotes would return items that contain any word included in that phrase.
That works fine except that before being able to use the form to its full potential, the user must learn the syntax. And yet, this kind of form cannot possibly offer the full power of SQL from a single text box. Applications try to address this limitation by providing advanced search forms.
An advanced form could be as simple as a group of related drop downs and text boxes, or as complex as a bulky collection of all input controls supported by the HTML specification:
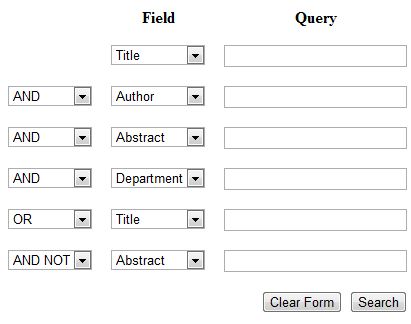
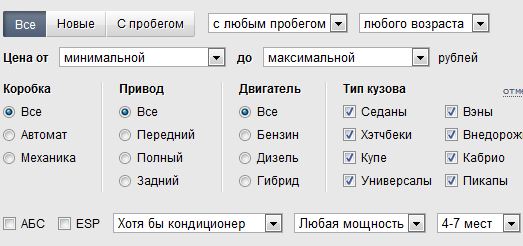
Even though these kind of forms are usually very complex, inside and out, they can't really predict all possible search needs that a user might have. A developer would have to invent some extreme and very hard to maintain UI control combinations and underlying server logic in order to create a UI that would be easy to use, but powerful enough to allow users to create, for example, the following where clause:
Get records where
date of birth is in the past of August 17, 1976 and
(
email address does not contain "domain" or
alcohol box is checked
)
and
(
(
home zip is "30040" and
gender is Male and
work city is not home city
)
or physician is equal to Stephen Lee
)
Well, Code Effects Software has already invented such a UI by introducing its Rule Editor. Now, to achieve the same goal, all developers need to do is to add it to the page, set its Mode to Filter, add a Search button, and add a simple code to handle its click event (a classic ASP.NET WebForms example is used; implementation examples on all .NET web platforms are available in our demo projects page):
<%@ Register assembly="CodeEffects.Rule.Editor.Asp"
namespace="CodeEffects.Rule.Asp" tagprefix="filter" %>
<div>
<filter:RuleEditor
ID="filterControl" runat="server"
SourceAssembly="DemoProject"
SourceType="DemoProject.Patient"
Mode="Filter" />
</div>
<div style="margin-top:10px;text-align:right;">
<asp:Button ID="btnSearch" runat="server" Text="Search"
CssClass="button" OnClick="SearchClicked" />
</div>
private void SearchClicked(object sender, EventArgs e)
{
if(this.filterControl.IsEmpty || !this.filterControl.IsValid)
{
this.someInfoLabel.Text = "The filter is empty or invalid";
return;
}
var dc = new YourEntityFrameworkDatabase();
var q = from p in dc.Patients.Filter(this.filterControl.GetRuleXml())
select new
{
p.PatientID,
p.FirtsName,
p.LastName,
p.Email
};
this.someGridViewControl.DataSource = q;
this.someGridViewControl.DataBind();
}
This code assumes that you have also added the someInfoLabel label and someGridViewControl grid view control to the page, and that the YourEntityFrameworkDatabase database with the Patient table is properly mapped in your project. By using this page, most business users are able to create the above filter in seconds, the same way they would create a business rule, even if they know nothing about Code Effects rules engine and how it works:
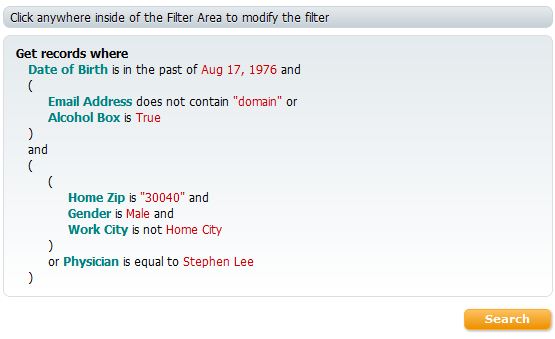
That's all it takes to create complex data filters and execute them against LINQ providers. Remember that in Code Effects, data filters are essentially evaluation type rules. Rules of execution type cannot be used as data filters.
When you set RuleEditor.Mode to Filter, Rule Editor hides the Toolbar and uses the filter-related built-in version of the Help XML while rendering labels and names on the client. All other settings and features of Code Effects in Filter mode work the same as documented in the Creating Business Rules section.
Rule Editor hides the Toolbar in Filter mode, because the most common use of any web-based filter is a "drive-by search": users visit a page, type search criteria into the search box, hit the Search button, and consume the results.
There could be situations where saving a filter is not only desirable, but actually required. In such cases, the use of Toolbar would greatly simplify filter management for users. Here are a few examples:
- A heavy reporting application that serves reports through long-running procedures. By saving a custom filter, the user could instruct the system's database to run a certain report with that filter applied, and send out a notification when the report is ready. Filters from all users could be stored as XML strings in a dynamic queue/table.
- An online store or auction that allows its users to store custom searches for items that are not yet (or no longer) available for sale. As new items arrive in the store, the system could run each new item through all relevant filters and notify the user that an item of interest just became available if any (or all) filters evaluated to True. The high performance of Code Effects' engine could help to make such system very fast and efficient.