Data Types in Code Effects
Code Effects business rules engine supports all .NET value data types (including nullables) and System.String except for the System.Guid and nullable enumerators. It also natively supports collections that implement the IEnumerable and IQueryable interfaces in both the busuness rules and rule-based data filtering features. Collection support is such an important area of Code Effects' UI that it has a separate topic dedicated to it.
Value Input Types
In Code Effects, the rule author can input values in three ways: manual input, field selection, or both. For numeric values, the manual input can be a value or a calculation. The input type of a particular field can be set by decorating it with the FieldAttribute class with its ValueInputType property set to one of the members of ValueInputType enumerator, with ValueInputType.All as the default value.
using System;
using CodeEffects.Rule.Common;
using CodeEffects.Rule.Attributes;
public class MySourceObject
{
public MySourceObject() { }
[Field( DisplayName = "My String")]
public string MyString { get; set; }
// Disallow calculation input for all numeric fields for now
[Field(ValueInputType = ValueInputType.Fields,
AllowCalculations = false, DisplayName = "My Decimal 1")]
public decimal MyDecimal1 { get; set; }
[Field(AllowCalculations = false, DisplayName = "My Decimal 2")]
public decimal MyDecimal2 { get; set; }
[Field(ValueInputType = ValueInputType.User,
AllowCalculations = false, DisplayName = "My Int 1")]
public int MyInt1 { get; set; }
}
If we were to set this class as a source object, we would see that MyDecimal1 field accepts only other numeric fields as its value, because its ValueInputType is set to Fields:
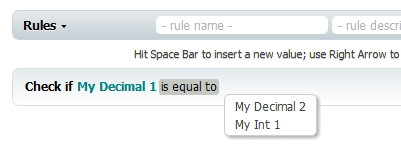
The MyDecimal2 field accepts both user input and other numeric fields as its value because of its default setting of ValueInputType.All:
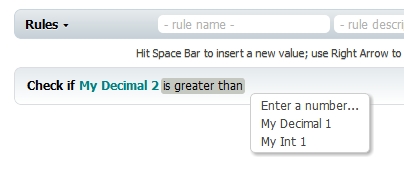
And the MyInt1 field accepts only manual input from rule authors because of its value of ValueInputType.User:
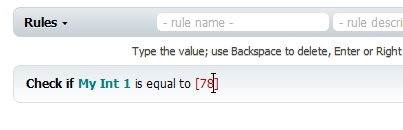
The ValueInputType works the same way for all value types, not only numeric. What's unique about the numeric type is its ability to accept calculated input. Enable it for any numeric field of the source object ...
[Field(ValueInputType = ValueInputType.All, AllowCalculations = true, DisplayName = "My Int 1")]
public int MyInt1 { get; set; }
... and Rule Editor will allow rule authors to input calculations as that field's value. (Set the ValueInputType to All to see all possible menu items for this particular field):
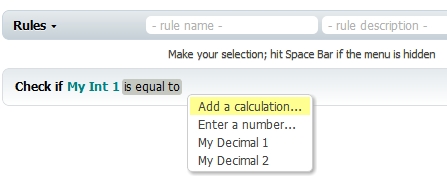
Calculations in Rule Editor are separated from the rest of the rule by {curly brackets}. Users don't need to input them; the editor does that automatically. Calculations support parentheses and simple math operators: multiplication, division, subtraction, and addition. Calculations may include numeric values as well as any numeric fields, as long as such fields are not marked to be excluded from all calculations. For example, an item ID has hardly anything in common with a price, tax, or shipping cost, and therefore, it makes sense to exclude it from all calculations by settings its IncludeInCalculations to False:
[Field(IncludeInCalculations = false)]
public long ID { get; set; }
This is what a calculation looks like in Rule Editor:
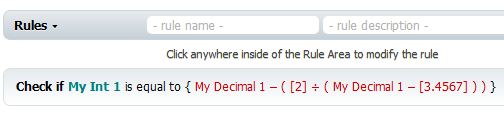
The only string field in our source object, MyString, accepts only manual input. This is not because of the value of its ValueInputType setting (it's set to the default value of All), but because there are no other string fields in the source object to select from:
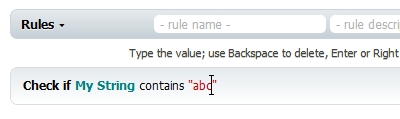
Implementations
(While the list below refers to field values, it is also true for in-rule methods, action parameters, and return values of string type as well. Use ParameterAttribute and ReturnAttribute to control the input values of those rule elements)
-
String Type. Values of System.String type are represented in Rule Area by <span> "value" element. It turns into a textbox control with its standard value input when the rule author enters a value. It turns back to <span> when user finishes input by clicking away or hitting the Enter key.
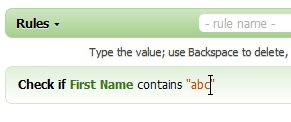
By decorating the string property of the source object with FieldAttribute you can control the case sensitivity and the maximum length of the text input. Use ParameterAttribute and ReturnAttribute to control the input values of those rule elements.
-
Numeric Types. All .NET numeric types are supported in Code Effects. Same as with string values, numerics are represented in Rule Area by <span> element. It turns into a textbox control with its standard value input when the rule author enters a value. It turns back to <span> when user finishes input by clicking away or hitting the Enter key. Input stops and Help String displays relevant warning messages if user types characters that are not compatible with the field's type.
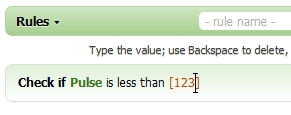
Code Effects Evaluator supports the comparison of values of different .NET numeric types, by performing type conversion automatically, at the time of rule evaluation. So, conditions such as IntegerValue is less than DoubleVaue are allowed. By decorating the numeric properties of the source object with FieldAttribute you can control the minimum and maximum values of their inputs. Depending on the exact .NET type of the field, Rule Editor automatically allows or disallows decimal point input.
-
Boolean Type. A value of System.Boolean type is represented in the Rule Area as a menu with two options, True and False:
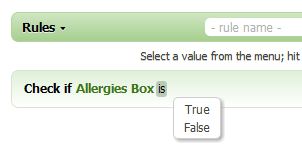
-
Enumerators. Enums are represented in the Rule Area as menus with their members as menu options:
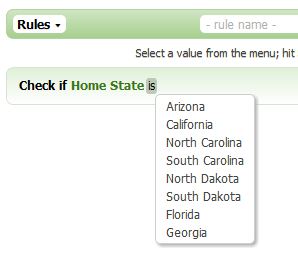
Use the ExcludeFromEvaluationAttribute to exclude any members of the enum form being used in the UI and, consequently, in the rules:
public enum State
{
Arizona,
California,
[EnumItem("North Carolina")]
NothCarolina,
[EnumItem("South Carolina")]
SouthCarolina,
[EnumItem("North Dakota")]
NorthDakota,
[EnumItem("South Dakota")]
SouthDakota,
Florida,
Georgia,
[ExcludeFromEvaluation]
Unknown
}
Nullable enumerators are not supported by Code Effects, and are ignored during the reflection of all source objects.
Code Effects business rules engine comes with a Dynamic Menu Data Sources feature that allows you to use dynamic key/value pairs as if they were enumerators. Read more about it in this topic.
-
DateTime Type. A value of System.DateTime type is represented in the Rule Area as a simple <span> element.
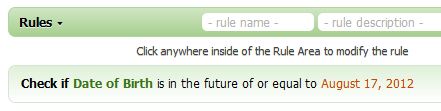
Rule authors can enter date values by using the built-in date picker (shown in the default format below):
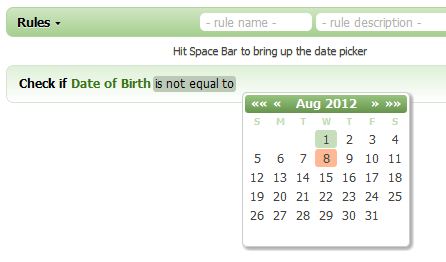
By decorating the DateTime properties of the source object with FieldAttribute, you can control the display format of the value as well as the layout of the picker. Rule Editor supports standard .NET date formatting, with a default format of "MMM dd, yyyy". Rule authors can pick the time as well as the date if the format contains the time portion. If the format contains no time, the value of the field that Evaluator actually uses during rule evaluation will contain the time of 12:00:00.000 AM. For example, if you set the format to "MM/dd/yy h:mm:ss tt":
[Field(DisplayName = "Date of Birth", DateTimeFormat = "MM/dd/yy h:mm:ss tt")]
public DateTime DOB { get; set; }
... the picker will look like this:
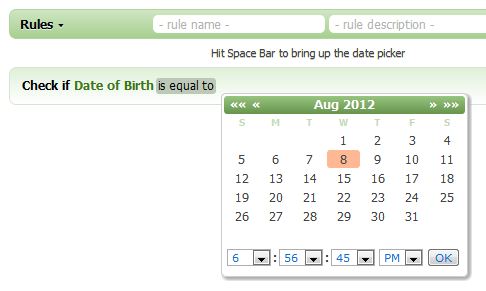
... and the value element will be displayed like this after the user picks the date:
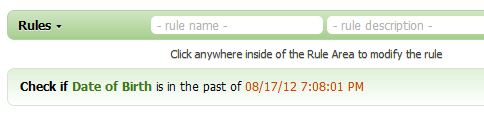
Here are a few more formats, just to complete the picture. The format that is used in most of Europe, "dd.MM.yyyy HH:mm", produces the following:
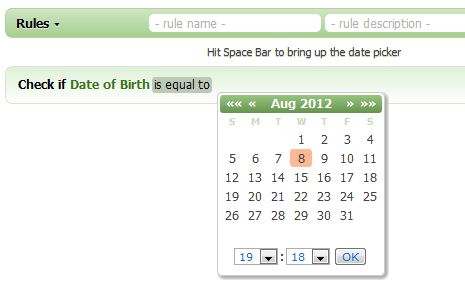
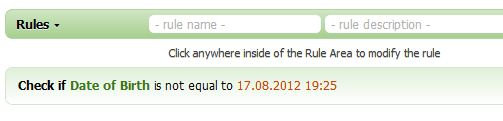
A format of "yyyy-MM-dd HH:mm:ss.fff" produces the following:
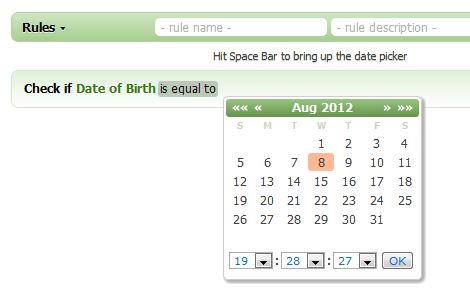
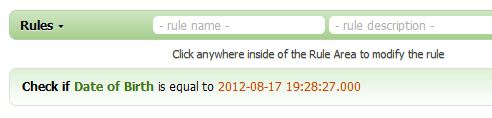
If the format contains no time portion, rule authors can pick a date just by clicking the desired day on the picker, otherwise the picker displays the OK button and the user must click it to confirm the selection that (s)he made.
-
TimeSpan Type. Support of the System.TimeSpan type in Code Effects allows rule authors to input a particular time without the date portion. TimeSpan value elements behave in a very similar manner to DateTime values, and are represented in the Rule Area as a simple <span> element.
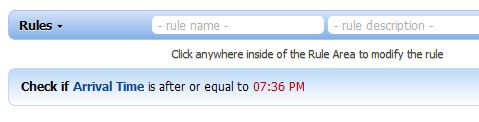
The format of the time picker differs from that of the date picker, and is shown in the default format below:
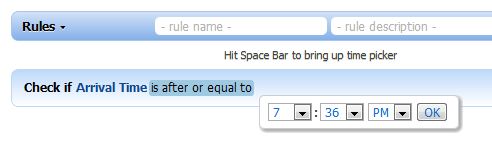
By decorating the TimeSpan-typed properties of the source object with FieldAttribute you can control the display format of the value as well as the layout of the time picker. As with DateTime types, Rule Editor supports standard .NET time formatting of TimeSpan values, with default format of "hh:mm tt".
-
The Guid Type. System.Guid type is not supported by Code Effects. The reason is simple: it's close to impossible to create a Guid-inputting control (a Guid picker) that would be intuitive enough for a non-IT individual. At first glance, it may seem obvious that a combination of five text boxes with some forced relation between them should do the job, but further research with focus groups shows that controls like these cause more confusion than they are worth. You can work around the Guid limitation by declaring a string field in your source object instead of (or in addition to) a Guid field.